As a beginner iOS developer, have you experienced the frustration of your app crashing unexpectedly due to memory issues? Trying to understand and fix them can be a real headache, especially when you’re just starting in the world of iOS development.
In this article, we’ll dive into the key concepts and best practices of iOS memory management that will help you easily navigate these challenges. By mastering these techniques, you’ll be able to create smooth-running, efficient apps that users will love.
So sit back, relax, and let us guide you through the ins and outs.
Importance of Memory Management
When you understand and effectively manage memory in your app, you can improve its performance, prevent crashes, and foster a better user experience.
iOS devices have limited memory resources, and as a developer, it’s crucial to ensure that your app uses these resources efficiently. Without proper memory management, your app may consume more memory than necessary, leading to performance issues such as sluggishness, freezing, and ultimately crashing.
Key Concepts in iOS Memory Management
How does iOS manage memory? These main concepts will help us understand the process better.
Automatic Reference Counting (ARC)
Automatic Reference Counting (ARC) is a fundamental concept that every beginner iOS developer should understand and utilize effectively. ARC is a feature provided by Apple in Xcode that automates the management of memory for your app, making memory management significantly easier and less error-prone.
In traditional manual memory management, developers had to allocate and deallocate memory for objects in their app explicitly. This manual process led to memory leaks, crashes, and other performance issues if not handled correctly. With ARC, you no longer need to worry about manually managing memory allocations and deallocations, as ARC takes care of this behind the scenes.
ARC works by keeping track of the number of references to an object in your app. When an object is created and assigned to a variable, ARC increments its reference count. When the object is no longer needed and the reference count drops to zero, ARC automatically deallocates the memory used by that object.
One key benefit of ARC is that it helps prevent common memory management issues like retain cycles and dangling pointers. It helps identify and break these cycles by allowing developers to use weak or unowned references when necessary.
Another advantage of ARC is that it frees developers from the burden of manual memory management in iOS Swift, allowing them to focus on writing clean, efficient code. This automated system saves time and reduces the likelihood of memory-related bugs and crashes in your app.
While ARC handles the majority of memory management tasks for you, it’s essential to understand how strong, weak, and unowned references work, as well as how to use them effectively in your code. By mastering the basics of ARC and familiarizing yourself with best practices for memory management in iOS development, you can create robust, stable apps that provide a seamless user experience.
Memory Management in iOS Swift
In Swift, memory management revolves around the concept of Automatic Reference Counting (ARC), which we discussed earlier. With ARC, the compiler automatically tracks and manages the memory used by your app, ensuring that objects are deallocated when they are no longer needed.
However, there are times when developers need to have a deeper understanding of iOS memory management in Swift, especially when dealing with complex data structures or handling resources like files or network connections.
Strong References
Strong reference cycles, also known as retain cycles, occur when two or more objects hold a strong reference to each other, creating a situation where the reference count of the objects never reaches zero, and thus they are never deallocated from memory.
This can lead to memory leaks, where memory is consumed unnecessarily and not properly released, causing the app to use more memory over time and potentially leading to app crashes due to memory exhaustion.
For example:
class Person {
var name: String
var car: Car?
init(name: String) {
self.name = name
}
deinit {
print("\(name) is being deallocated")
}
}
class Car {
var brand: String
var owner: Person?
init(brand: String) {
self.brand = brand
}
deinit {
print("\(brand) is being deallocated")
}
}
var john: Person?
var tesla: Car?
john = Person(name: "John")
tesla = Car(brand: "Tesla")
john?.car = tesla
tesla?.owner = john
In this example, the “john” instance of the Person class and the “tesla” instance of the Car class create a strong reference cycle by holding on to each other through their properties. Even if you set both variables to nil, the reference count of both objects will not reach zero because they are still holding a strong reference to each other.
According to the iOS memory management best practices, to break the strong reference cycle and prevent memory leaks, you can use weak or unowned references depending on the scenario:
– Use a weak reference when the referenced object can be deallocated and set to nil if needed.
– Use an unowned reference when the referenced object will always exist as long as the current object exists.
In the example above, you can break the strong reference cycle by using a weak reference for the car property in the Person class:
class Person {
var name: String
weak var car: Car?
init(name: String) {
self.name = name
}
deinit {
print("\(name) is being deallocated")
}
}
By using weak references carefully and understanding how strong reference cycles can occur, you can avoid memory leaks and ensure that your app’s memory is managed efficiently.
Unowned References
Memory management in iOS Swift features another type of reference similar to weak references but with a key difference. They do not keep a strong hold on the referenced object. Unlike weak references, unowned references assume that the referenced object will always exist (i.e., it will not be deallocated) for as long as the referring object exists.
Here are some key points:
- Unowned references are used when you are certain that the referenced object will not be deallocated before the object holding the reference.
- Unowned references are created using the unowned keyword.
- Unowned references do not increase the reference count of the referenced object. This means that if the referenced object is deallocated, accessing the unowned reference will result in a runtime crash.
- These references are useful when you have a one-to-one relationship between objects, such as a parent-child relationship where the child object never outlives the parent object.
An example to demonstrate the use of unowned references:
class Parent {
var name: String
var child: Child?
init(name: String) {
self.name = name
}
deinit {
print("\(name) is being deallocated")
}
}
class Child {
var name: String
unowned var parent: Parent
init(name: String, parent: Parent) {
self.name = name
self.parent = parent
}
deinit {
print("\(name) is being deallocated")
}
}
var john: Parent?
var child: Child?
john = Parent(name: "John")
child = Child(name: "Alice", parent: john!)
In this example, the Child class has an unowned reference to the Parent class. Since the relationship is such that a child object will never exist without a parent object, it is safe to use an unowned reference.
According to iOS memory management best practices, it’s important to use unowned references only when you are certain that the referenced object will always exist. If it can be deallocated, it’s better to use weak references to avoid potential crashes.
Resolving Reference Cycles
Also known as retain cycles, they occur when two or more objects hold strong references to each other. This creates a situation where the memory management system can’t deallocate any objects. The situation leads to memory leaks and inefficient memory usage in your application.
There are several techniques to resolve reference cycles:
- Weak references: Use them when one object should not keep a strong hold on another object. Weak references do not increase the reference count of the referenced object, so they automatically become nil when the referenced object is deallocated. This breaks the reference cycle and allows the objects to be deallocated properly.
- Unowned references: They are used when one object should always exist as long as another object exists. Unlike weak references, unowned ones assume that the referenced object will never become nil, so accessing a deallocated object will lead to a runtime crash.
- Capture lists: Use capture lists to break reference cycles in closures. In a closure, if you capture self strongly, you can create a reference cycle. With a capture list and capturing self weakly or unowned, you can break the reference cycle and prevent memory leaks.
- Weak self in closures: Use weak self or [weak self] in closures to capture the self reference weakly. This prevents strong reference cycles when self is captured in a closure. Remember to unwrap self safely before using it in the closure to avoid runtime crashes.
- Deinit method: Apply the deinit method to perform cleanup and break reference cycles. In the deinit method of a class, set properties to nil or break strong reference cycles manually to ensure proper deallocation of objects.
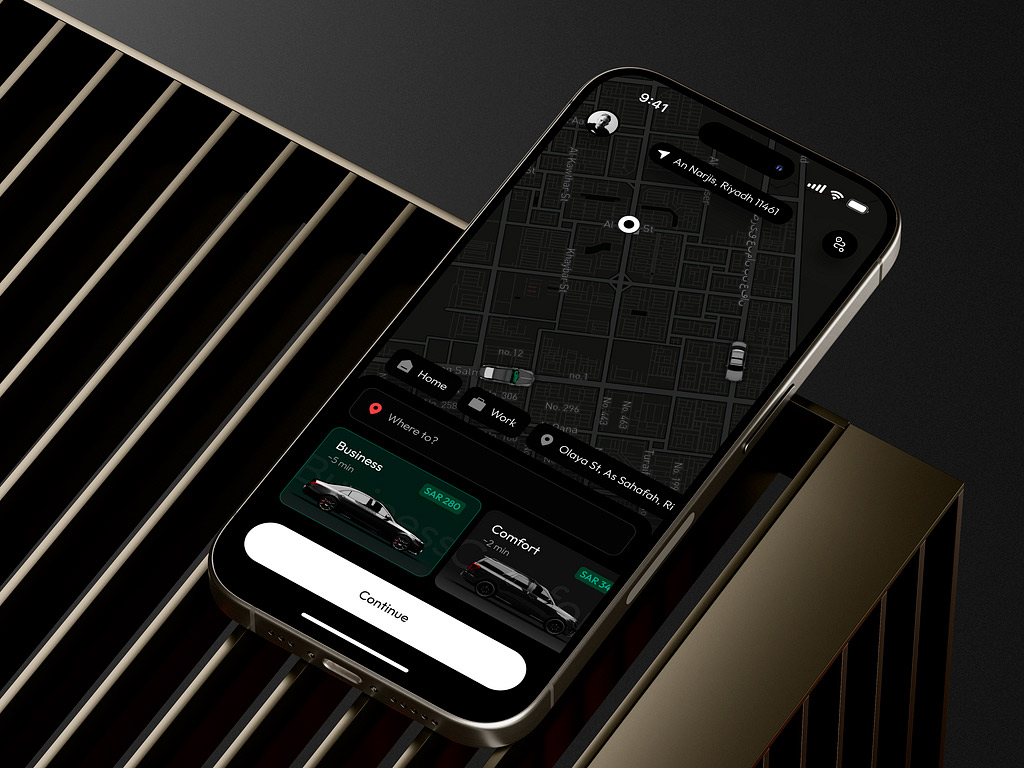
High-End Chauffeur Service App by Shakuro
Common Memory Management Issues
Here are some common issues in iOS memory management that you may encounter and how to address them:
- Retain cycles: Also known as strong reference cycles, they occur when two or more objects hold strong references to each other, preventing them from being deallocated. To avoid retain cycles, use weak or unowned references when capturing self in closures and be mindful of object relationships to prevent strong reference cycles.
- Memory leaks: When objects are not deallocated properly, it leads to a gradual increase in memory usage and potential crashes. To prevent memory leaks, make sure to release strong references to objects when they are no longer needed, use weak references when appropriate, and properly manage the lifecycle of objects.
- Over-retention: Over-retention happens when an object continues to hold a strong reference to another object even after it is no longer needed. This can lead to the retain cycles and memory leaks. Be careful when retaining objects and release strong references when they are no longer needed to prevent over-retention.
- Accessing deallocated objects: It can result in a runtime crash. To avoid this issue, use weak references or unowned references to prevent strong reference cycles and crashes when accessing objects that may have been deallocated.
- Circular references: These occur when two or more objects hold strong references to each other in a circular manner, creating a retain cycle. To break circular references, use weak or unowned references appropriately and carefully manage object relationships to avoid retain cycles.
- Not implementing deinit method: The deinit method is called when an object is about to be deallocated and is a good place to perform cleanup tasks and break reference cycles. Make sure to implement the deinit method in your classes to release resources and break strong reference cycles to prevent memory leaks.
iOS Memory Management Best Practices
Here are some key tips to help you optimize memory usage in your iOS applications:
- Use ARC (Automatic Reference Counting): iOS leverages ARC to manage memory automatically, reducing the need for manual effort. ARC automatically tracks references to objects and deallocates them when they are no longer needed, simplifying memory management for developers.
- Avoid retain cycles: Retain cycles occur when two or more objects hold strong references to each other, preventing them from being deallocated. To avoid retain cycles, use weak or unowned references when capturing self in closures and be mindful of object relationships to prevent strong reference cycles.
- Release strong references when they are no longer needed: Make sure to release strong references to objects when they are no longer needed to prevent memory leaks and optimize memory usage. Use tools like Instruments to monitor memory usage and identify any retained objects that need to be released.
- Use weak or unowned references when capturing self in closures: This allows the closure to capture a reference to self without creating a retain cycle, ensuring that objects are deallocated properly.
- Implement the dealloc method: In addition to using ARC, implement the deinit method in your classes to perform cleanup tasks and break strong reference cycles. The deinit method is called when an object is about to be deallocated and is a good place to release resources and break retain cycles.
- Profile and debug memory usage: Use Xcode’s Instruments tool to profile memory usage in your app and identify memory issues such as leaks and high memory consumption. Analyze memory allocations, leaks, and overall memory footprint to optimize memory management and improve app performance.
Memory Management Tools
There are several powerful tools available to help you with memory management in iOS Swift. Here are some key tools that you should become familiar with:
- Instruments: It is a powerful profiling tool by Xcode that allows you to monitor and analyze various aspects of your app’s performance, including memory usage. You can track memory allocations, identify memory leaks, and measure overall memory usage in real-time. By running your app through Instruments, you pinpoint memory issues and optimize your memory management strategies.
- Memory Graph Debugger: The Memory Graph Debugger in Xcode helps visualize object relationships and memory allocations. It provides a graphical representation of the memory graph, allowing you to see object references and detect retain cycles that can lead to memory leaks. You can gain insights into your app’s memory structure and identify areas for improvement.
- Leak Detection: Xcode includes a built-in tool for detecting memory leaks in your app. By running the Leak Detection tool, you can identify objects not being deallocated properly and causing memory leaks. It highlights the issues and provides information on the objects leaking memory, helping you to address and fix them.
- Static Code Analysis: With static code analysis, Xcode can detect common memory-related errors such as retained cycles, unbalanced retain/release calls, and memory leaks. Utilizing static code analysis can help you catch memory management issues early in the development process and ensure a more stable and efficient app.
- Third-Party Libraries: In addition to Xcode’s built-in tools, there are several third-party libraries and tools available for memory management and performance optimization in iOS development. Libraries like Facebook’s FBMemoryProfiler and Google’s LeakCanary provide advanced memory profiling capabilities and can help you identify memory issues in your app more effectively. Consider exploring these third-party tools to enhance your memory management practices and improve your app’s performance.
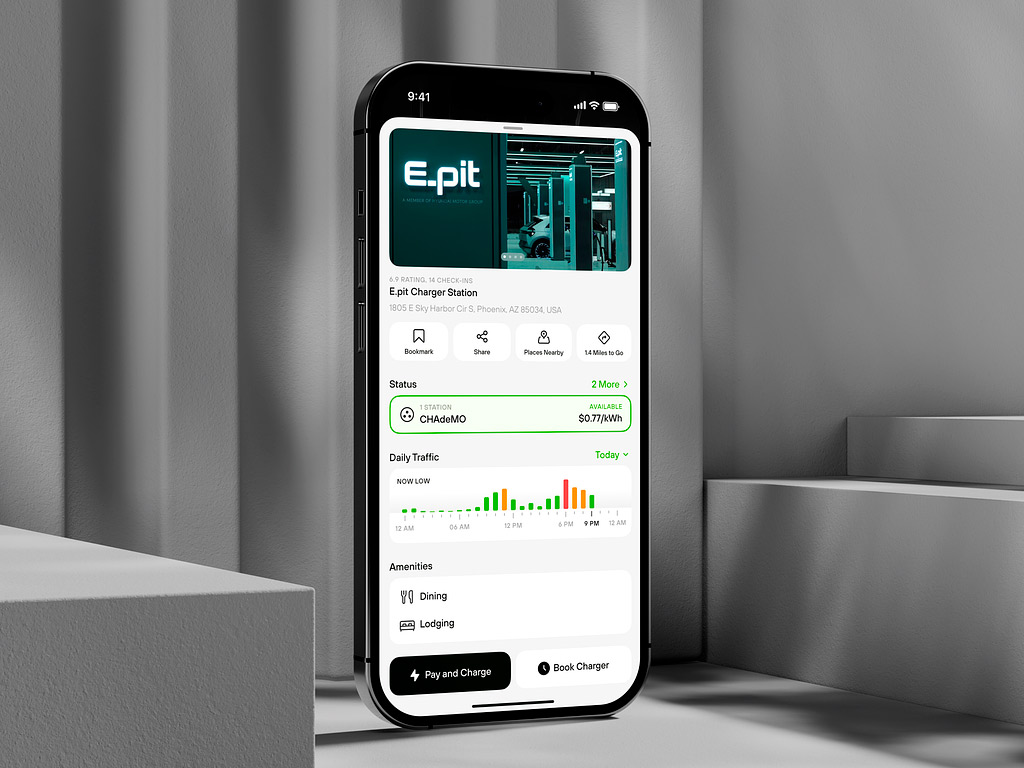
Electric Vehicle Charger Mobile App by Conceptzilla
Android vs iOS Memory Management
Both platforms have their own memory management systems that you must be familiar with to optimize your apps effectively. Here is an overview of some key differences between the systems:
- Manual vs Automatic Memory Management:
– iOS: Memory management is handled automatically through ARC. With ARC, the compiler inserts code into your app’s source code, managing memory allocations and deallocations for objects. As a developer, you don’t need to explicitly allocate or deallocate memory, making memory management easier and less error-prone.
– Android: The OS uses a manual memory management system where developers are responsible for explicitly allocating and freeing memory using methods like allocate() and free(). This manual approach requires you to carefully manage memory allocations and deallocations, making it more prone to memory leaks and memory-related issues if mishandled.
- Garbage Collection:
– iOS: It does not have a traditional garbage collection system like Android. Instead, iOS uses ARC to track object references and automatically release memory when objects are no longer needed. ARC helps prevent memory leaks by managing object ownership and releasing memory when objects are no longer referenced.
– Android: This one uses a garbage collection system to reclaim memory by identifying and collecting unused objects. The garbage collector runs periodically to scan memory for unreferenced objects and free up memory. While garbage collection can help manage memory automatically, it can also introduce performance overhead and potential pauses in app execution during garbage collection cycles.
- Memory Leaks:
– iOS: With ARC, memory leaks are less common compared to Android. ARC automatically manages object references and deallocates memory when objects are no longer needed, reducing the risk of memory leaks caused by unused objects retaining memory.
– Android: The manual memory management and the garbage collection system can lead to memory leaks if you do not release memory properly. Memory leaks occur when objects are not properly released and continue to consume memory, causing performance issues and potential crashes in the app.
- Memory Optimization:
– iOS: It provides tools like Instruments for profiling and analyzing memory usage in your app, helping you identify memory issues and optimize memory management strategies. You can monitor memory allocations, identify memory leaks, and optimize memory usage for improved app performance.
– Android: Similarly, Android offers tools like Android Profiler and LeakCanary for memory profiling and leak detection. These tools help developers analyze memory usage, detect memory leaks, and optimize memory management practices to enhance app performance and stability.
In terms of Android vs iOS memory management, both operating systems have their ups and downs. So pick the one that is suitable for your current project.
Conclusion
Memory management is a critical aspect of iOS development that you must grasp to create efficient and high-performance mobile applications. Understanding the principles of memory management in iOS, including Automatic Reference Counting (ARC) and memory optimization tools like Instruments, is essential for preventing memory leaks, improving app performance, and ensuring a smooth user experience.
Remember to regularly monitor and profile your app for memory issues to minimize memory-related problems and provide users with a seamless and responsive app experience. With a solid foundation in iOS memory management, you can elevate your skills and create impactful mobile applications that stand out in the competitive app market.
Do you need to build a functional iOS app? Reach out to us and let’s work together on your next project.