For those who prefer to listen rather than read, this article is also available as a podcast on Spotify.
Contents:
As a startup founder or a beginner developer, you’re juggling a million things at the same time. Deadlines are breathing down your neck, half-finished features are piling up, and there is probably some bug lurking in the code that just won’t go away, no matter what you do. There are days you look at the code and think, “How does this even work?”
Well, sometimes software development is close to building a house while someone keeps moving the foundation. It’s messy, stressful, and sometimes you’re making progress only to realize the first floor is already going down. Again. I’ve been there, looking at the enormous task pool my team has to finish before the release. But hey, over time, I’ve learned that there are ways to make this whole process less chaotic.
Most of the pain points come from skipping over the basics proven by time. Or worse, not knowing that software development best practices exist in the first place. You might be thinking, “I’ll figure it out as I go,” but trust me, that approach is a road to nowhere. Like winging it on a road trip without checking if your car has enough gas. You’ll end up stranded somewhere inconvenient, with a long way to your destination.
So here’s where the good stuff comes from: best practices. I know what you’re thinking—“Oh great, another list of rules that will mess up my familiar process .” But don’t regard these tips as rigid commandments etched in stone. They are tools, tricks, and habits that actually save you time and headaches.
If you’re ready to turn your coding chaos into something smoother or understand the development process better, keep reading.
Introduction to Software Development Best Practices
Why Best Practices Matter
Well, what if you just trash all these tips and go with the flow? Just design, code, fix, and release, what can be so difficult? High chances are that a lot of things will go wrong.
Impact on Code Quality and Maintenance
Let’s imagine the following situation. You’ve built an app. It works fine for now, but you cut a few corners along the way because, hey, deadlines. You didn’t write tests because “it’s not that complicated,” and documentation? Who has time for that? Fast forward six months, and suddenly users are reporting bugs left and right. Your once-manageable codebase now feels like a labyrinth designed by someone who hates you. And that person is you from the past.
As I said, software development is inherently messy. Things break. Requirements change. New people join the team—or worse, old people leave, taking their brainpower with them. Without software development life cycle best practices in place, it’s easy to lose control. And losing control over your own project is one of the most frustrating feelings out there.
So yeah, the practices help your team create high-quality code and maintain that quality even after the release. When your workflow is streamlined, you can focus more on creativity and innovation instead of firefighting. Want to add a new feature? Go for it—you’ve got a solid foundation to build on. Over time, those small investments pay off big-time.
Business Benefits: Cost Reduction and Faster Delivery
When you’re knee-deep in deadlines, it’s tempting to slap together a solution that works right now and worry about the consequences later. But those quick fixes almost always come back to haunt you, and they bring friends. Friends with names like “technical debt,” “rework,” and “unexpected bugs.” Those uninvited guests aren’t cheap. Rework alone can account for up to 40-50% of development costs in poorly managed projects.
Software engineering best practices help you avoid these hidden expenses. Writing clean, maintainable code, for instance, might take a bit more time upfront, but it saves you from hours (or days) of debugging down the road. Similarly, automated testing ensures that new features don’t break old ones, reducing the need for costly manual checks every time you make a change.
You might think speed and quality are at odds with each other, like choosing between pizza and tacos when you’re hungry. Both are tasty, no question about that! However, Agile practices actually help you move faster. How? Well, developing a product without structure is navigating a city without street signs. Best practices act as those street signs, guiding you toward your destination without unnecessary detours.
Take version control systems like Git, for example. They allow multiple developers to work on the same project simultaneously without stepping on each other’s toes. Need to roll back a bad commit? No problem—it’s just a few clicks away. Without proper version control, untangling conflicting changes can turn into a nightmare that slows everyone down.
Prevention Over Cure
Writing unit tests, for example, catches issues before they make it to production. Fixing a bug during development is exponentially cheaper than fixing it after release. Studies show that catching a defect post-release can cost 100x more than addressing it during the design phase. Automated testing frameworks like Jest or Selenium help nip these problems in the bud, saving both time and money.
Following best practices ensures your codebase is flexible enough to grow with your business. Need to add a new feature? With modular, well-structured code, you can slot it in without breaking existing functionality. Without that foundation, scaling becomes a Herculean task, often requiring a complete rewrite, which is neither fast nor cheap.
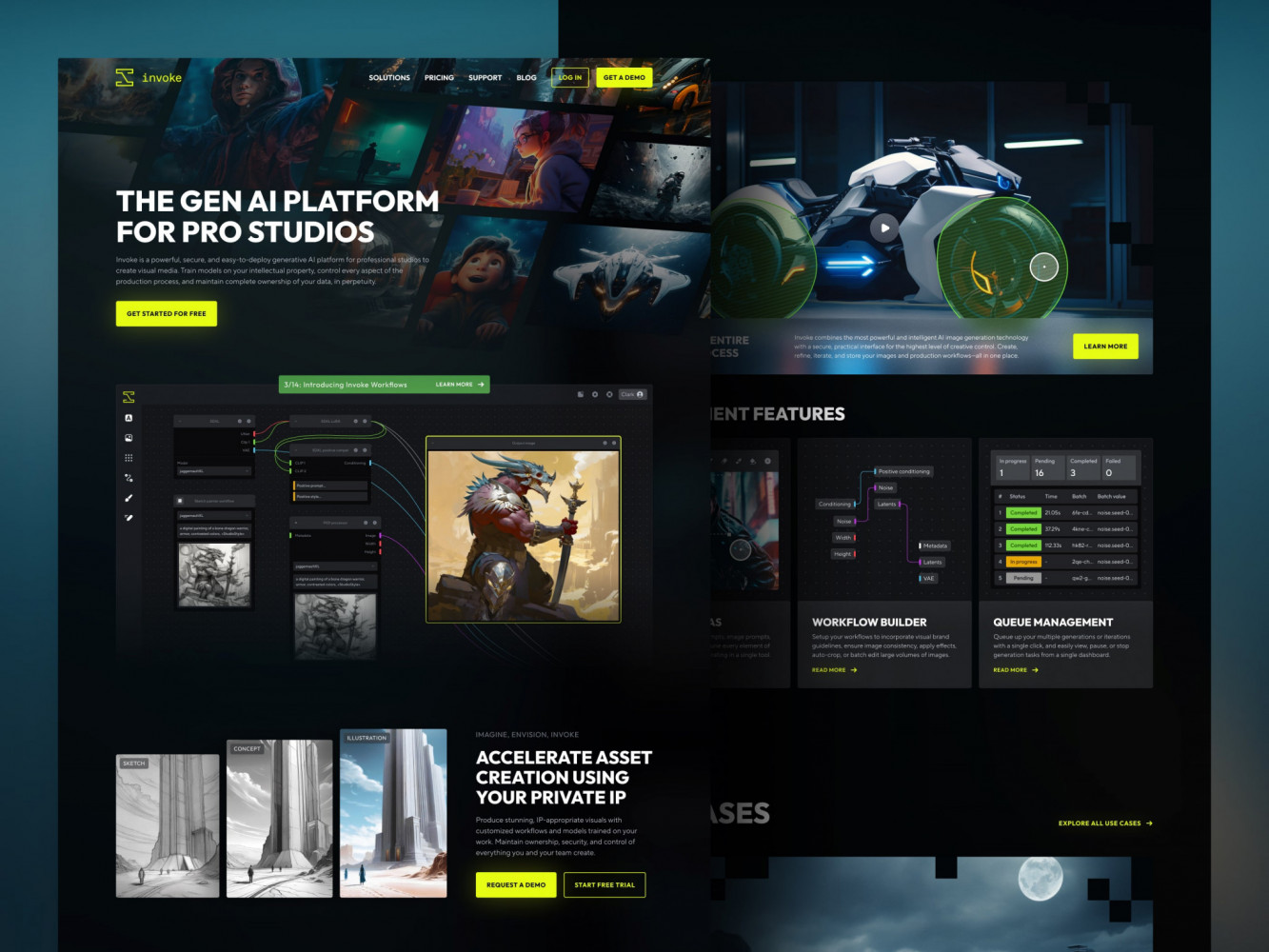
Landing Page Redesign for Creative Production by Shakuro
Foundational Development Standards
Now that you know the importance of these practices, time to actually dive into the topic. What are the best software development practices?
Code Quality & Maintainability
Terrible code might be acceptable in the short term, but in the long term, it’s a haven for bugs, inefficiencies, and frustration. And trust me, no one desires to trudge through a “code swamp” where each minor modification is reminiscent of trying to walk through quicksand. There are tried-and-tested principles and practices that help make things simpler.
DRY and YAGNI: Less Is More
You’ve probably heard of DRY (Don’t Repeat Yourself) and YAGNI (You Aren’t Gonna Need It). These two principles might sound simple, but they turn the tables if you apply them consistently.
DRY
Let’s take an example of copying and pasting the same block of code ten times. Sure, it works—for now. But when you need to modify that logic, what then? You’ll have to hunt down each instance, which takes time and is error-prone. DRY encourages you to write reusable components or functions, so modifications are made in a single location. Cleaner, smarter, faster—that’s the path of DRY.
YAGNI
Remember an overwhelming urge to include all the shiny features simultaneously? These software development best practices are for you. It is all about not over-engineering things. Just because you can possibly add a feature does not mean you have to. Unnecessary complexity increases maintenance costs and makes your code less readable. It should be lean, with today’s issues fixes, not tomorrow’s maybes.
Code Style Guides and Naming Conventions
Clever code isn’t always clear code. When it comes to writing maintainable software, clarity trumps cleverness every day.
That’s where code style guides and naming conventions come in. They provide a way to introduce consistency into your codebase so that it’s easier for any person to read and contribute to. Whatever you decide to use, the most crucial thing is to pick a standard and stick to it.
And as far as naming conventions go, they are all about communication. Expressive names for your variables, functions, and classes are a must because they tell you exactly what they do.
Modular Design: Think LEGO, Not Spaghetti
Well-written code is a modular thing, meaning that it’s broken up into smaller, independent chunks. Easily understandable and reusable, just like LEGO. The benefits are countless, but I’ll name just a few:
- Easier debugging: If something goes wrong, you can isolate the issue to a specific module instead of having to dig through the entire codebase.
- Scalability: Adding new functionality is a breeze because modules are self-contained and won’t interfere with each other.
- Reusability: Need the same functionality elsewhere in your application? Just insert the existing module instead of reinventing the wheel.
Your code is a LEGO set. Each piece serves one purpose, and you can snap them together to form complex structures without needing to start from scratch. Contrast with spaghetti code, where all the things are tangled up together, and good luck untangling it when something goes wrong.
Documentation: Your Safety Net
No matter how clean your code is, documentation is important. It’s leaving a breadcrumb trail for yourself and anyone else who might end up on the project. As a software engineering best practice, at least provide:
- Inline comments: Explain why something is being done, not what it does. Future You will thank you.
- README files: Provide a project overview, setup instructions, and any important notes.
- API documentation: If your project involves APIs, properly document endpoints, parameters, and expected responses.
Honestly, I used to despise writing documentation. I felt it was make-work. But when I had to pass the task to another team member, questions and explanations consumed most of our time. So yes, it is worth spending some time writing everything down instead of wasting hours in the future. Not to mention something might just slip your mind.
Security-First Development
A single vulnerability can lead to data breaches, financial losses, damage to reputation, and even legal consequences. And no one wants to be the person who must sit down with their boss (or, even worse, their clients) and explain why sensitive information was compromised.
Secure Coding Standards
Two of the most well-respected frameworks are:
OWASP (Open Web Application Security Project)
It’s a treasure trove for developers looking to learn about and steer clear of common weaknesses. Their flagship resource, the OWASP Top Ten, provides the most critical security threats to web applications today, like injection attacks, broken authentication, and cross-site scripting (XSS). OWASP also offers detailed guidance on how to thwart these threats, which makes it a resource that developers can’t do without.
CERT Secure Coding Standards
Developed by the CERT Division of Carnegie Mellon University, these standards focus on language-specific secure coding rules. Regardless of whether C, Java, Python, or other languages, CERT provides prescriptive guidelines to avoid vulnerabilities like buffer overflows, faulty error handling, and insecure API usage.
To follow them is to study the principles behind them and apply them by habit. For example, always sanitize user input (one of the most critical OWASP guidelines) to prevent malicious users from injecting malicious code. Or follow CERT’s recommendation to use safer functions instead of older ones prone to vulnerabilities.
Regular Security Testing
Even with the best practices in security testing for software development in place, no system is ever 100% bulletproof. That’s why regular security testing is non-negotiable.
- Static Application Security Testing (SAST): Analyze your source code for vulnerabilities before it’s deployed. For example, SonarQube or Checkmarx scans your codebase and flags potential issues, such as hardcoded passwords or unsafe dependencies. SAST catches problems early, when they’re easier to fix.
- Dynamic Application Security Testing (DAST): This is the reverse of SAST; it tests your application while it’s running. It simulates real attacks to discover vulnerabilities like SQL injection or XSS. Tools like Burp Suite or OWASP ZAP are ideal for this. DAST is a fire drill that makes sure your defenses remain solid while under attack.
- Penetration testing (Pentesting): This is the ultimate stress test. Ethical hackers try to exploit weaknesses in your system to see how far they can penetrate. Pentesting gives you a clear picture of your app’s robustness and tells you where you need to beef up. It does not come cheap, but it is worth every penny if it saves you from a breach.
- Dependency scanning: Software has a high reliance on third-party libraries and frameworks, but these introduce vulnerabilities. Scanning your dependencies regularly using tools such as Dependabot or Snyk keeps you ahead of patches and updates.
Defense in Depth
These software development security best practices involve having several layers of defense, so if one fails, others remain. For instance:
- Encrypt sensitive information both in transit and at rest.
- Have strong authentication mechanisms, such as multi-factor authentication (MFA).
- Have role-based access control (RBAC) so that users only access what they need.
- Keep your systems updated and patched regularly to shut down known vulnerabilities.
It is just like wearing a helmet and a seatbelt when riding a motorbike. Each provides an additional layer of protection, making the likelihood of catastrophe less probable.
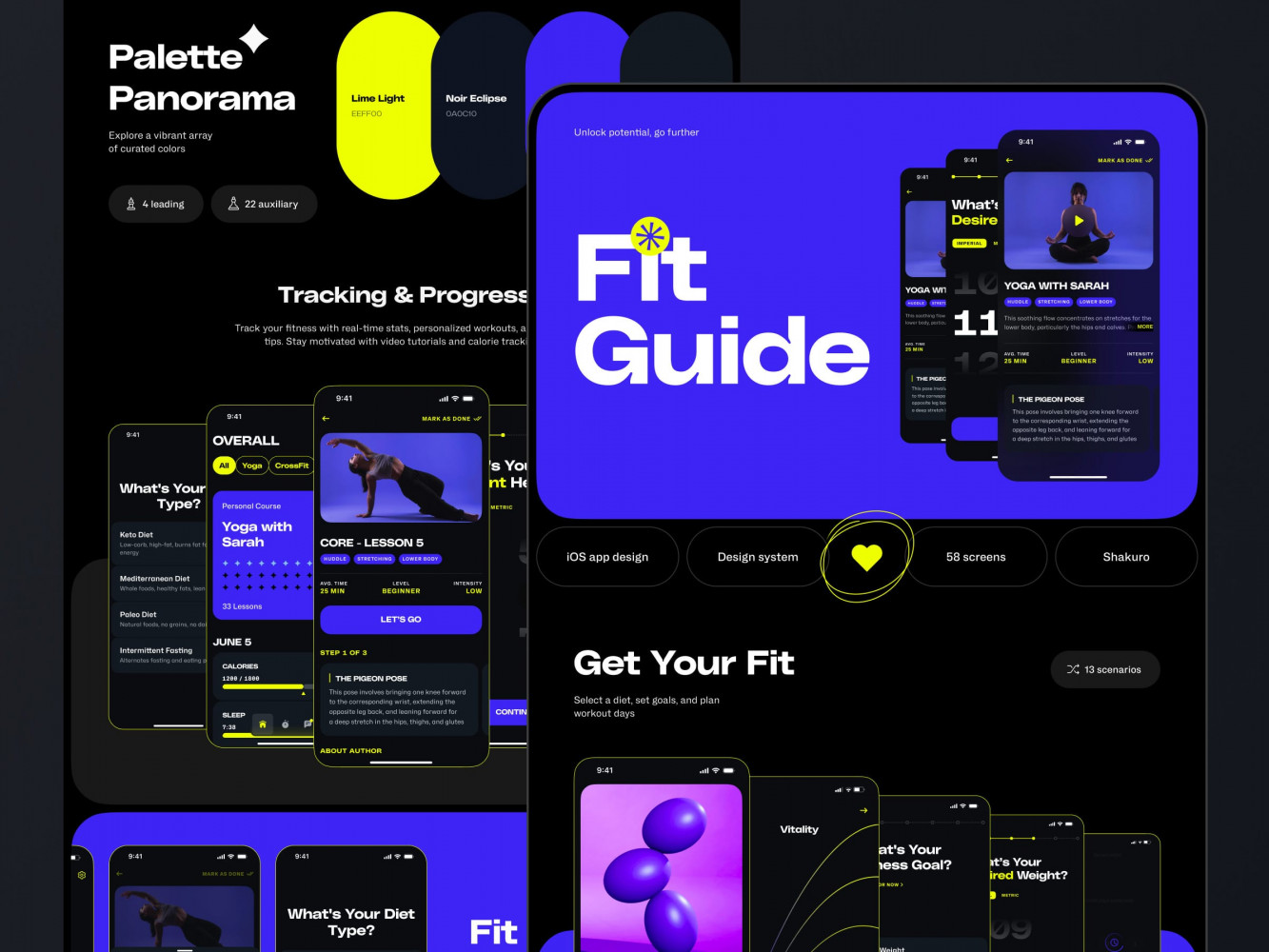
Website Design for a Yoga & Wellness Platform by Shakuro
Agile & Collaborative Practices
The Waterfall methods are not magic bullets: there are some cases when they fail. If you have no idea what a Waterfall is, then here is a brief explanation. You spend months laying out all the details first, then development, then testing, and finally deployment. It’s a linear, sequential process where each phase of the project needs to be completed before you can proceed to the next. It’s like climbing a staircase—you can’t skip steps or go back once you’ve moved forward. However, sometimes requirements change halfway through, and chaos is unleashed. The logical structure just can’t adapt.
Instead of taking months to produce results and proceed to the next action, Agile practices slice work into manageable chunks, with the teams reacting fast and shipping features in the meantime. You get feedback along the way, make adjustments as needed, and have everyone walk away happy.
Agile Methodologies
Iterative Development and Sprints
At the heart of this approach is iterative development —the idea that software is created in cycles of building, testing, and iteration. Each iteration (or sprint) delivers a small, functional piece of the product, which can be viewed and iterated upon based on feedback.
Here’s why it’s important:
- More rapid feedback cycles: By making small updates in small cycles, you can get feedback from users earlier and more often. This allows you to create something that humans actually need, rather than what you think they need.
- Fewer risks: If something becomes fouled up, it is better to go looking for and fixing issues with a small release than with a huge rollout. It’s like repairing a dripping faucet instead of reworking the entire plumbing system.
- Continuous improvement: With each iteration, your team becomes smarter and smarter. Processes become more refined, code quality increases, and communication becomes smoother with time.
Sprints are the pillars of numerous Agile frameworks, particularly Scrum. A sprint is a concise, time-boxed duration (typically 1-4 weeks) when a team concentrates on finishing a predefined list of activities. The vision at the termination of the sprint is to produce a potentially shippable product increment.
Why these software development best practices put things back in line:
- Specific objectives: Each sprint starts with a planning session where the team makes a commitment to specific objectives. This eliminates any ambiguity and keeps everyone moving in the same direction.
- Standups: Short daily meetings (approximately 15 minutes) keep everyone in sync. Everyone describes what they did yesterday, what they are doing today, and if they are blocked on anything. It’s an efficient but brief way of raising issues before they become unmanageable.
- Retrospectives: At the end of each sprint, the team reflects on what went well and what didn’t. These retrospectives foster continuous learning and adaptation.
Sprints gain momentum. There’s something so fulfilling about marking off items and watching actual progress at the end of each cycle. And stakeholders just love having regular check-ins to see how things are progressing.
Feature Flags: Roll Out with Confidence
They allow you to toggle features off or on without deploying new code. That is, you can deploy incomplete features to production under a flag with controlled rollouts and no risk.
Why are feature flags such effective software engineering best practices?
- Incremental roll-out: Instead of rolling out a feature to all users at once, you can roll it out to a subset of your users first. If it doesn’t work, you can just flip the flag off without having to roll back the entire deployment.
- A/B testing: Want to test two versions of a feature to see which performs better? Feature flags make it easy to split traffic between different implementations and measure the results.
- Trunk-based development: Feature flags are a perfect fit with trunk-based development, in which all the developers push to one branch. Instead of long-lived feature branches (which create merge conflicts), you push changes into the main branch and hide incomplete features behind flags.
Cross-Functional Teams
And yet another feature of Agile is cross-functional teams. Instead of isolating developers, designers, and testers into their respective departments, Agile practices bring everybody together to work on the process. It speeds up decision-making and enables you to consider everybody’s voice from the very start.
For instance, including QA engineers in the initial design phase avoids the introduction of usability issues at all, even before a line of code has been written. Similarly, inclusion of a product owner in the team keeps business goals topmost. When people are aligned toward the same objective, magic is achieved.
Team Collaboration
The Agile approach shines when it comes to team work, breaking down silos and building an environment where everyone works together towards shared goals. You don’t sit aside all alone coding in isolation, dealing with static mockups thrown by designers, while testers find bugs at the last minute. The methodology brings all your team members together into one solid unit.
Code Reviews and Pair Programming
If you’ve ever written code, you know how simple it is to miss something: a crafty bug, a performance issue, or even a naming inconsistency. That’s where code reviews come in.
These secure coding standards involve having another developer (or developers) review your code before it’s merged into the master branch. This does a few things:
- Catching errors early: An extra pair of eyes can spot errors that you might have overlooked. Like proofreading an essay, you’re too familiar with your own work to notice every typo.
- Bringing consistency: Code reviews enable coding standards and style guides to be adhered to, making the entire codebase consistent. No more “mystery code” that reads completely differently from the rest of the project.
- Knowledge sharing: By having someone read your code, they learn from your solution to the problem, and vice versa. In the long term, this builds up shared knowledge within the team.
- Mentorship opportunities: Junior devs learn through criticism by more experienced developers, and seniors keep their minds sharp having to explain themselves.
Ever heard the saying “two heads are better than one”? That’s the principle of pair programming, where two programmers sit at the same workstation and collaborate. One is the driver, who writes the code, while the other is the navigator, who reads the code as it’s being written and thinks more about the larger strategy.
Why pair programming rules:
- Higher-quality code: Errors are detected as and when they occur with two individuals collaborating, and solutions are more sound. A debugger is inherent.
- Rapid problem-solving: Two brains tackling a difficult issue come up with ideas faster and create novel solutions that neither would have thought of individually.
- Knowledge transfer: A great way of onboarding new members or sharing knowledge across the team. For example, a junior developer who is working with a senior engineer can pick up shortcuts and best practices that they would otherwise not learn.
- Improved communication: Pairing encourages open communication and strengthens bonds within the team.
That said, pair programming is not possible for all tasks. It requires focus and effort, so it is best reserved for complex or high-risk features. If you use these software development life cycle best practices wisely, they are a powerful technique for collaboration and skill development.
Shared Code Ownership
In older models of development, it is not uncommon for developers to “own” parts of the codebase. While this might seem at first to be effective, it leads to bottlenecks. What happens when the “owner” of a module goes on vacation—or, even worse, leaves the company? Suddenly, no one else knows how to modify or maintain that part of the system.
Agile reverses this model with collective code ownership. Under this philosophy, the entire team owns the codebase. Anyone can contribute to any part of the project, provided they follow pre-established standards and guidelines.
Here’s why collective code ownership is revolutionary:
- Less risk: When someone leaves, the project doesn’t grind to a halt. There are multiple people familiar with every part of the system, so there’s continuity.
- More flexibility: Programmers don’t need to work on the same modules forever. They change tasks, which makes things interesting and prevents burnout.
- Collective responsibility: When the code belongs to everyone, there’s more pride and responsibility. People are less likely to cut corners because they know others will be reading their code.
Shared ownership does require trust and discipline, naturally. You need to have clear coding standards, thorough documentation, and regular communication for consistency.
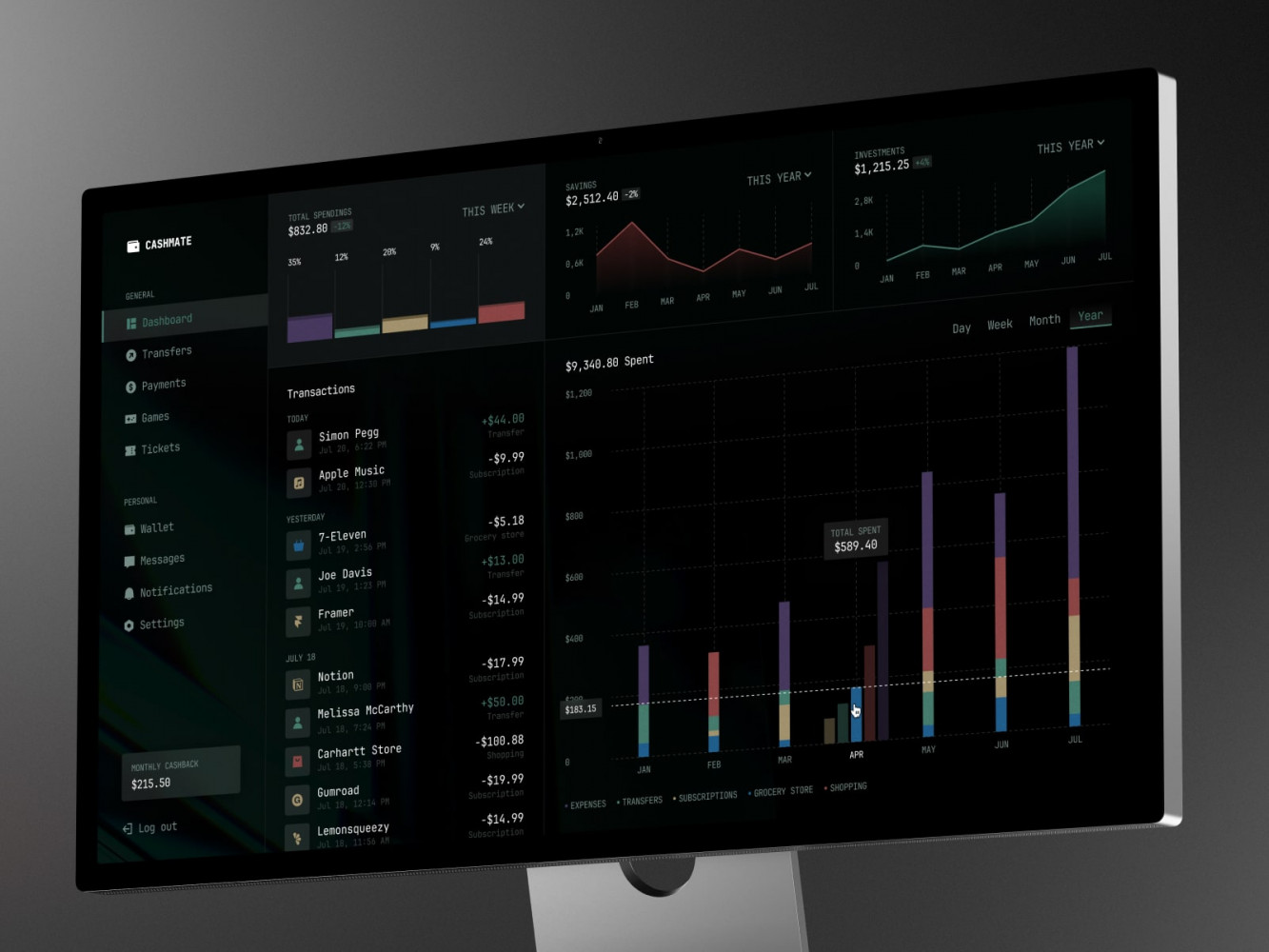
Finance Management Web Dashboard by Conceptzilla
Technical Excellence
Obviously, without proper testing, your software is a house of cards waiting to topple. You need to verify that your code does what you think it will, works consistently under load, and can be changed without collapsing. So, tests are necessary. But there is one “but”.
For software development best practices, manual testing alone just doesn’t cut it anymore. The sheer complexity of applications and the speed at which features are delivered make automated testing an absolute necessity. Spice it up with metrics like test coverage, and you’ve got a robust strategy for building confidence in your codebase.
Automated Testing (Unit, Integration, E2E)
No matter how much knowledge and experience a senior developer has, there is a fair limit to their time and stamina. Machines don’t get tired. So, you test your code quickly, repeatedly, and relentlessly without relying on humans to click through all possible scenarios.
Unit testing
It verifies individual components or functions independently. It is a kind that makes sure small pieces of your code are right for specific conditions. For example, if you have a sales tax calculation function, a unit test would verify whether it gives the correct output for various inputs.
Unit tests catch bugs early, often even before they’ve entered the larger system. Since they’re fast and specific, it’s your best bug-fighting companion that you can run frequently during development. Leverage frameworks like JUnit (Java), pytest (Python), or Jest (JavaScript) and write tests for edge cases, invalid inputs, and expected functionality.
Integration testing
In these software development best practices, you verify different modules or services communicate with one another as expected. For instance, if your app talks to a database, an integration test might verify the execution of queries and fetching of data.
The majority of bugs happen at the interface between components. Integration tests uncover issues like incompatible APIs, wrong data formats, or broken dependencies. Isolate the interaction being tested by mocking external systems where necessary. Tools like Postman (API testing) or Testcontainers (database testing) can be extremely helpful.
End-to-End (E2E) testing
They mimic real user interactions by going through the entire application flow—from login to checkout to logout. They operate at the highest level, where all the components in the system integrate together seamlessly.
E2E is a safety net for your most critical workflows. When something breaks in production, these tests are typically the first line of defense. Use tools like Selenium, Cypress, or Playwright for automating browser interactions. Focus on key user journeys rather than trying to cover all possible paths.
Test Coverage Metrics
It measures how much of your code is executed by automated tests. While coverage isn’t a silver bullet, it’s a decent measure of how thoroughly your tests exercise your codebase.
There are three types of coverage:
- Line: Measures the percentage of lines of code executed during tests.
- Branch: Tracks whether all decision paths (i.e., if/else statements) are tested.
- Function: Ensures every function or method is called at least once.
Such high test coverage gives you confidence that most of your code has been tested. Having said that, 100% coverage is not always possible—or desirable. Parts of your code are so simple they don’t require tests, while others entail too much complex setup that wouldn’t be worthwhile.
A realistic goal would be aiming for a balance. A good rule of thumb is 80-90% coverage for the core logic and critical APIs. For less critical components, having lower coverage could be acceptable.
CI/CD & Deployment
This one, from the software development best practices checklist, is about merging code updates into a shared repository on a regular basis, where automated builds and tests verify everything works as expected. The goal is to identify issues early, before they snowball into bigger problems.
At its essence, CI/CD streamlines the processes between writing code and releasing it to customers. It decreases human effort, reduces errors, and ensures your software is in a state of release at all times.
Continuous Integration Best Practices
- Commit early, commit often: Instead of waiting days or weeks to commit your changes, you commit small, incremental changes to the main branch. This keeps the codebase fresh and reduces the likelihood of merge conflicts. I’d compare it to saving in a video game—you don’t want to risk losing hours of work because you didn’t click “save.”
- Automate tests and builds: With every code push, your CI pipeline should automatically build the app and run unit, integration, and even basic E2E tests. Jenkins, GitHub Actions, GitLab CI/CD, or CircleCI make this a breeze.
- Fail fast, fix faster: If a build or test fails, halt everything until the problem has been fixed. This may be harsh-sounding, but it keeps bad code from propagating and corrupting other areas of the system. A broken CI pipeline is like a smoke alarm—it’s bothersome, but it’s there for a purpose.
- Make the pipeline fast: No one likes waiting for builds to finish. Optimize your pipeline through parallel tests, cache dependencies, and bypass unnecessary work. Receive feedback in minutes, not hours. You must move fast because if you wait too long, you’ll lose momentum and productivity.
- Enforce code quality gates: Use static analysis tools (e.g., SonarQube) and linters to enforce secure coding standards and mark potential issues before they reach the production phase. This is a safety net where only clean, maintainable code goes through.
Small Batch Deployments
Development today is moving towards small batch deployments, where you deploy small, incremental updates on a regular basis. There are several advantages to this practice:
- Lower risk: By deploying one or two changes at a time, you can more readily spot and fix errors if something goes wrong. Compare that with a big release where lots of features are interdependent—like untying garlands.
- More streamlined feedback loops: With small deployments, you can get user feedback sooner. If a feature isn’t taking hold or isn’t usable, you can course correct without having spent money on additional development.
- Simpler rollbacks: If something goes wrong after a deployment, it is much easier to roll back a minor change than to undo a whole release. Feature flags (which I’ve discussed above) help here too, enabling you to switch features on or off without redeploying.
- Increased team morale: Shipping also gives the team a sense of accomplishment. Instead of spending months to see their hard work mirrored, you are able to savor little triumphs on a regular basis.
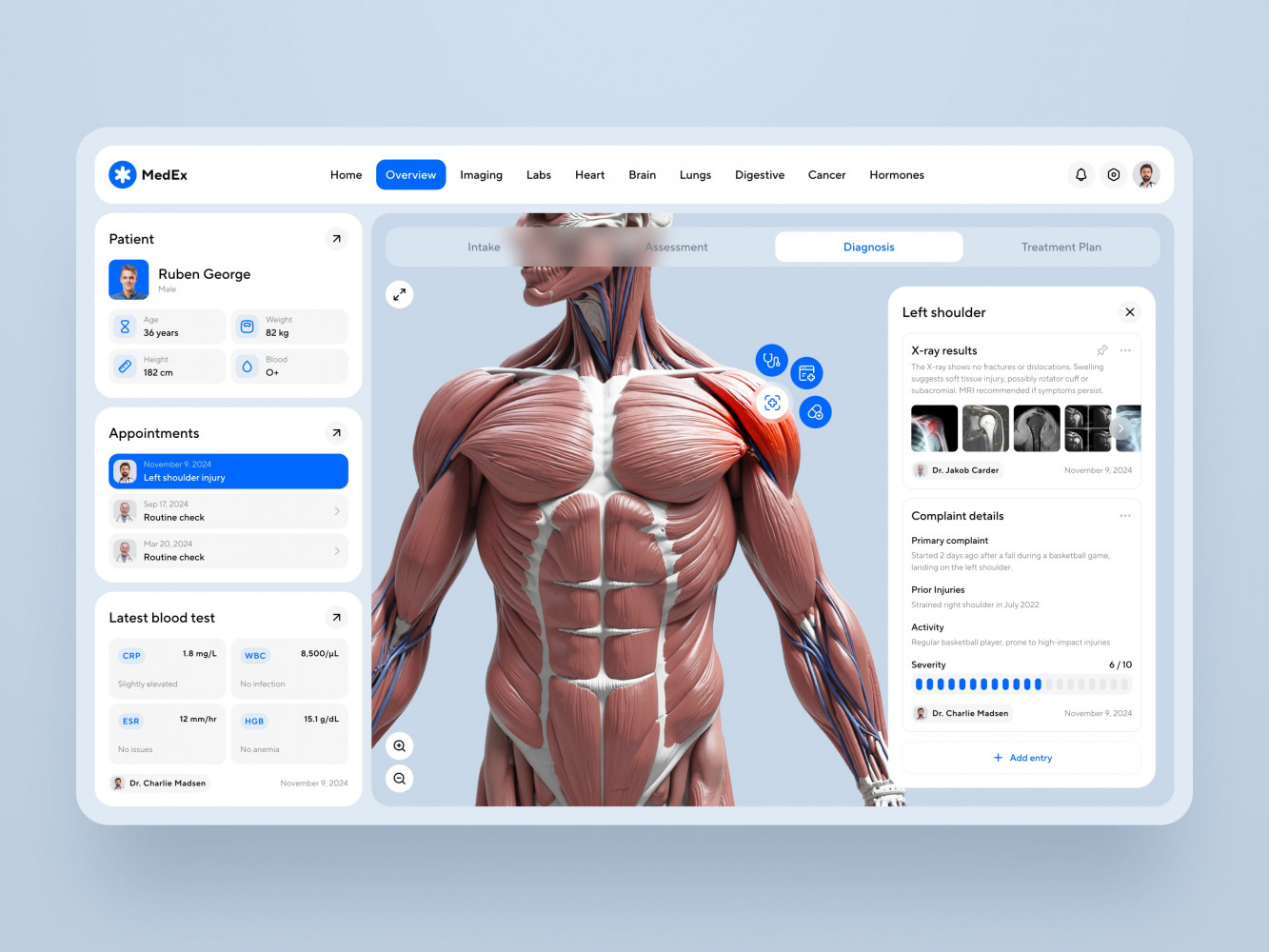
Medical Practice Management Software Dashboard Design by Shakuro
Tools That Help You Adopt Best Practices
I’ve already mentioned some useful tools for different cases along the way, but I’ll elaborate on them once again in case you skipped some sections.
Code Management: Keeping Your Codebase Healthy
Version Control Systems (VCS)
Without VCS, collaboration becomes a nightmare, and seeking changes is like finding a needle in a bundle of hay. So it’s another brick in the foundation of the whole development process. Remove it and it all will go down.
What you can check out:
- Git: The gold standard of version control. You track changes, create branches, and collaborate with your team in sync.
- GitHub/GitLab/Bitbucket: These Git-hosting platforms give you additional features like pull requests, issue tracking, and CI/CD pipelines. Which one to use? If you have an open-source project, opt for GitHub. GitLab is good at DevOps features, and Bitbucket pairs well with Atlassian products like Jira.
Code Review Tools
Review is one of the software development best practices, but with the right instruments, it can be done much faster. And you can’t skip it because it turns into an essential component of quality assurance. These tools make the process easier:
- GitHub pull requests: Integrated in GitHub, pull requests allow teams to review code changes, comment, and approve merges.
- Gerrit: For managing code reviews in large projects, this one is an extremely efficient tool. No more wasting time on staring at endless lines.
- Crucible: Part of the Atlassian suite, Crucible provides fine-grained code review processes and integrates with Jira.
Static Analysis & Linting Tools
These tools catch possible issues before reaching production:
- SonarQube: Scans for bugs, security vulnerabilities, and technical debt in code and provides actionable data to improve quality.
- ESLint/Prettier: For TypeScript/JavaScript projects, ESLint promotes coding rules and Prettier ensures uniform format.
- Checkmarx/Snyk: Focus on security through scanning the code for vulnerabilities and outdated dependencies.
Automation: Doing More with Less Effort
Automation is the magic sauce that facilitates Agile and DevOps practices. Using these software development life cycle best practices, you cut out mundane tasks and thus focus on creating something new instead of human busywork.
Continuous Integration/Continuous Deployment (CI/CD)
I’ll refresh your memory: CI/CD pipelines automate the steps from coding to deploying to users. These kinds of tools are invaluable:
- Jenkins: Highly versatile open-source tool for building CI/CD pipelines.
- GitHub Actions: Native to GitHub, allows you to automate workflows in your repository.
- GitLab CI/CD: Integrated into GitLab, offers end-to-end automation from testing through deployment.
- CircleCI: Fast and scalable, CircleCI is ideal for cloud-native apps.
- Azure DevOps: Provides a broad set of tools for CI/CD, project management, and more.
Infrastructure as Code (IaC)
Automating infrastructure setup ensures consistency and reduces human errors:
- Terraform: An extremely popular IaC tool for cloud resource creation and management.
- Ansible: Simplifies configuration management and app deployment.
- AWS CloudFormation/Azure Resource Manager: Vendor-specific cloud infrastructure management software on AWS and Azure, respectively.
Testing Automation
You don’t have again all eternity to manually verify everything. Automated testing tools reduce time and improve reliability:
- JUnit/TestNG: For Java unit testing.
- pytest: A cross-purpose testing system for Python.
- Selenium/Cypress/Playwright: For end-to-end browser testing.
- Postman: Excellent for API testing and documentation.
Monitoring: Staying Ahead of Problems
Even well-written code will fail in production. Monitoring solutions give you visibility into the health of your system so that you can detect and repair issues before users are impacted.
Application Performance Monitoring (APM)
APM tools monitor your application’s performance in real-time:
- New Relic: Provides detailed insights into application performance, database queries, and user experience.
- Datadog: Merges monitoring, logging, and tracing to provide a complete picture of your system.
- AppDynamics: Emphasizes business-critical metrics in addition to technical performance.
Logging and Error Tracking
Logs and error reports are priceless when debugging and making reliability improvements:
- ELK Stack (Elasticsearch, Logstash, Kibana): A powerful three-pack for centralized logging and analysis.
- Splunk: An enterprise-grade tool for log aggregation, searching, and visualization.
- Sentry: Watches for errors in real-time and provides detailed stack traces for easy fixing.
Infrastructure Monitoring
Watch servers, containers, and other infrastructure components:
- Prometheus: An open-source monitoring system with strong alerting capabilities.
- Grafana: Works in tandem with Prometheus to build stunning dashboards for metric visualization.
- Nagios: A tried-and-true tool for monitoring network services and infrastructure.
Collaboration & Project Management
Collaboration is at the very top of the software development best practices checklist. If the team is unmotivated, the work will snowball, bugs will appear, and the deadline will be the sword of Damocles. So you definitely need tools for effortless communication between teams, with project management tools to keep everyone on the same page when it comes to goals and priorities.
Communication Platforms
- Slack: The go-to team communication platform, with integrations for almost every development tool.
- Microsoft Teams: Bakes together chat, video meetings, and document collaboration, best in enterprise environments.
Project Management Tools
- Jira: A heavy-duty option for Agile teams, with sprint planning, backlog management, and reporting.
- Trello: Simple to use and visual, Trello is great for smaller teams or less complex projects.
- Asana: Task management and workflow automation oriented, with a clean interface.
Knowledge Sharing
- Confluence: Best suited for the production of internal documentation and knowledge bases.
- Notion: An all-purpose workspace for notes, documents, and collaboration among teams.
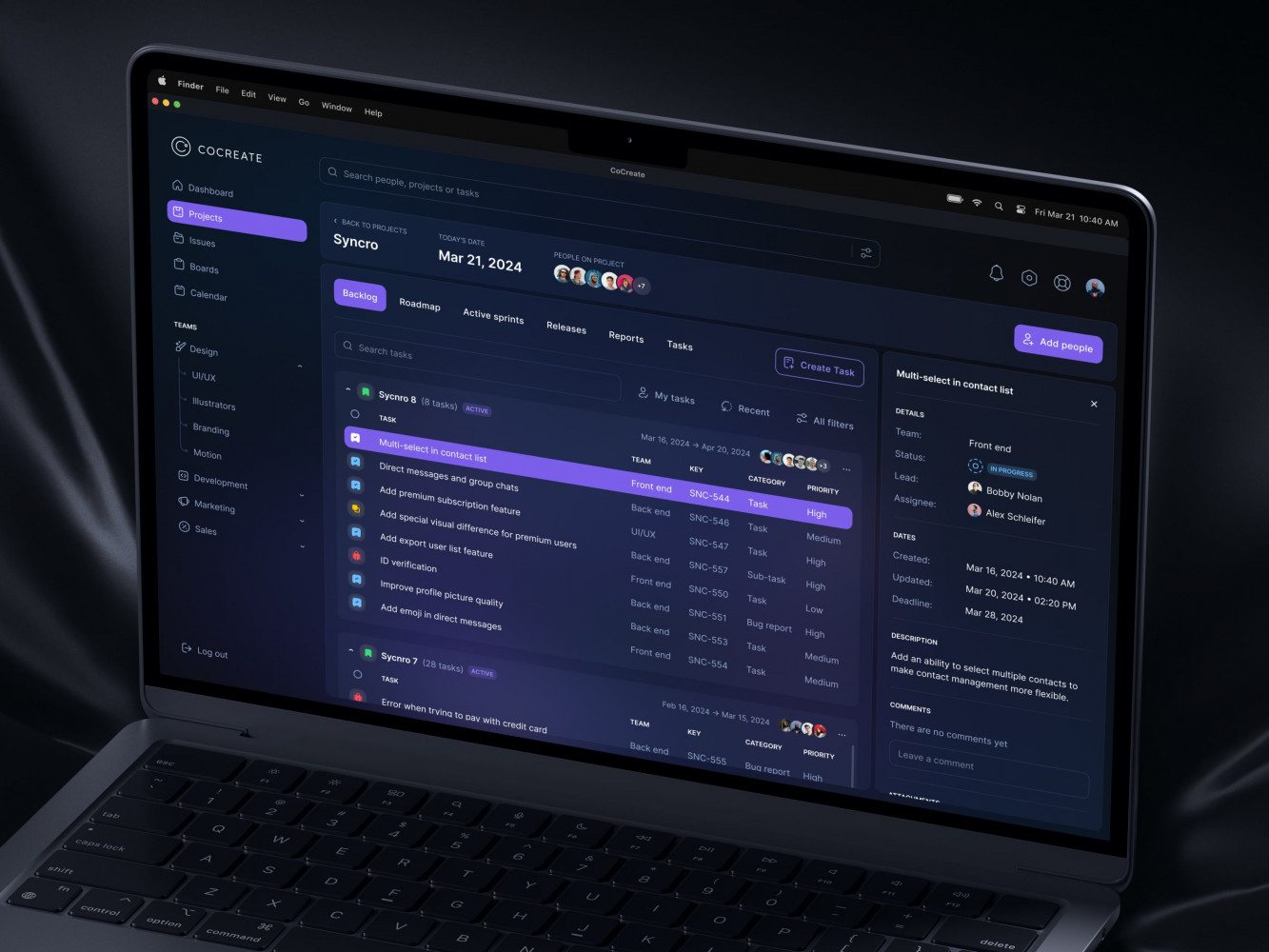
UI Design for a Task Management Tool by Shakuro
Avoiding Common Pitfalls
There are some pitfalls that are technical, some that are organizational, but all have one thing in common: they’re avoidable if you know what to do and how to do it.
Technical Debt Accumulation: The Silent Project Killer
It’s like junk food—tastes great in the moment, but harm it causes awaits you to catch up later on. All of the shortcuts, the hacks, the compromises we make along the way to make it happen on time or be out there in a hurry as soon as possible. These “cheats” accumulate over time and make the codebase more brittle, harder to work on, slower to change, and buggy.
Why it happens:
- Pressure to deliver: People usually want it fast rather than right, so they rush you to deliver it sooner.
- Lack of planning: When there is no proper architecture or secure coding standards, there are strong possibilities that you write dirty code.
- Neglecting refactoring: When you miss rewriting code for improvement because you are too busy adding new features.
How to avoid it:
- Admit it exists: Recognize technical debt as a real issue. Track it explicitly, like financial debt.
- Refactor regularly: Prioritize refactoring within every sprint. A bit of small improvement prevents debt from becoming unmanageable.
- Enforce code quality: Use tools like linters, static analyzers, and enforced code reviews to detect bad patterns early.
- Engage stakeholders: Educate non-technical stakeholders about the future cost of technical debt. Frame it as an investment in future efficiency.
I had worked on a project where we let technical debt go for months due to “we didn’t have time.” Naturally, our velocity went down, bugs rose, and morale sank. When we did take a break to clean up, it took weeks. Therefore, repaying technical debt ahead of time saves much more work than leaving it.
Scope Creep: The Never-Ending Project
It happens when the goals of a project expand beyond the original plan without adjusting timelines or resources. What starts as a simple app can quickly morph into a sprawling platform if you’re not careful.
Why it happens:
- Unclear requirements: Vague specifications leave room for interpretation and feature bloat.
- Stakeholder pressure: Clients or managers keep adding “just one more thing” without considering the impact.
- Lack of boundaries: Some developers, especially juniors, are eager to please and say “yes” to every request instead of pushing back.
How to avoid it with software development best practices:
- Define clear goals: Start with a well-documented scope that outlines exactly what will (and won’t) be delivered.
- Use Agile practices: Break work into small, manageable chunks and prioritize ruthlessly. Only add new features after completing the current scope.
- Say no gracefully: Politely push back on unreasonable requests by explaining trade-offs and impacts on timelines.
Neglecting User Feedback: Building for Nobody
It’s easy to fall into the trap of building features based on assumptions rather than actual user needs. However, in this case, you risk creating an app that nobody really needs.
Why it happens:
- Overconfidence in ideas: You believe you know what users really want without validating their assumptions.
- Delayed testing: Waiting until the final stages to gather feedback means costly rework if the product misses the mark.
- Ignoring negative feedback: Dismissing criticism instead of using it to improve.
How to avoid it:
- Engage users early: Conduct interviews, surveys, and usability tests during the design phase.
- Iterative releases: Ship small updates frequently and gather feedback after each release.
- Listen actively: Treat user feedback as gold—it’s the best source of insight into what truly matters.
Over-Engineering: Solving Problems That Don’t Exist
Sometimes, the biggest enemy of simplicity is ambition. Quite often, developers strive to build overly complex solutions for problems that don’t yet exist—or may even never arise. Over-engineering is another root of evil that the software development life cycle best practices aim to purge.
Why it happens:
- Ego-driven development: You want to showcase your skills, even if it’s unnecessary.
- Fear of future changes: Anticipation is good, but not for every possible scenario. It bloats the system.
- Misaligned priorities: Focus shifts from delivering value to building technically impressive features.
How to avoid it:
- Stick to YAGNI: Remember—you aren’t gonna need it. Build only what’s required today.
- Keep it simple: Favor straightforward solutions over clever ones. Simplicity scales better.
- Prioritize business value: Always ask, “Does this add value for users?”
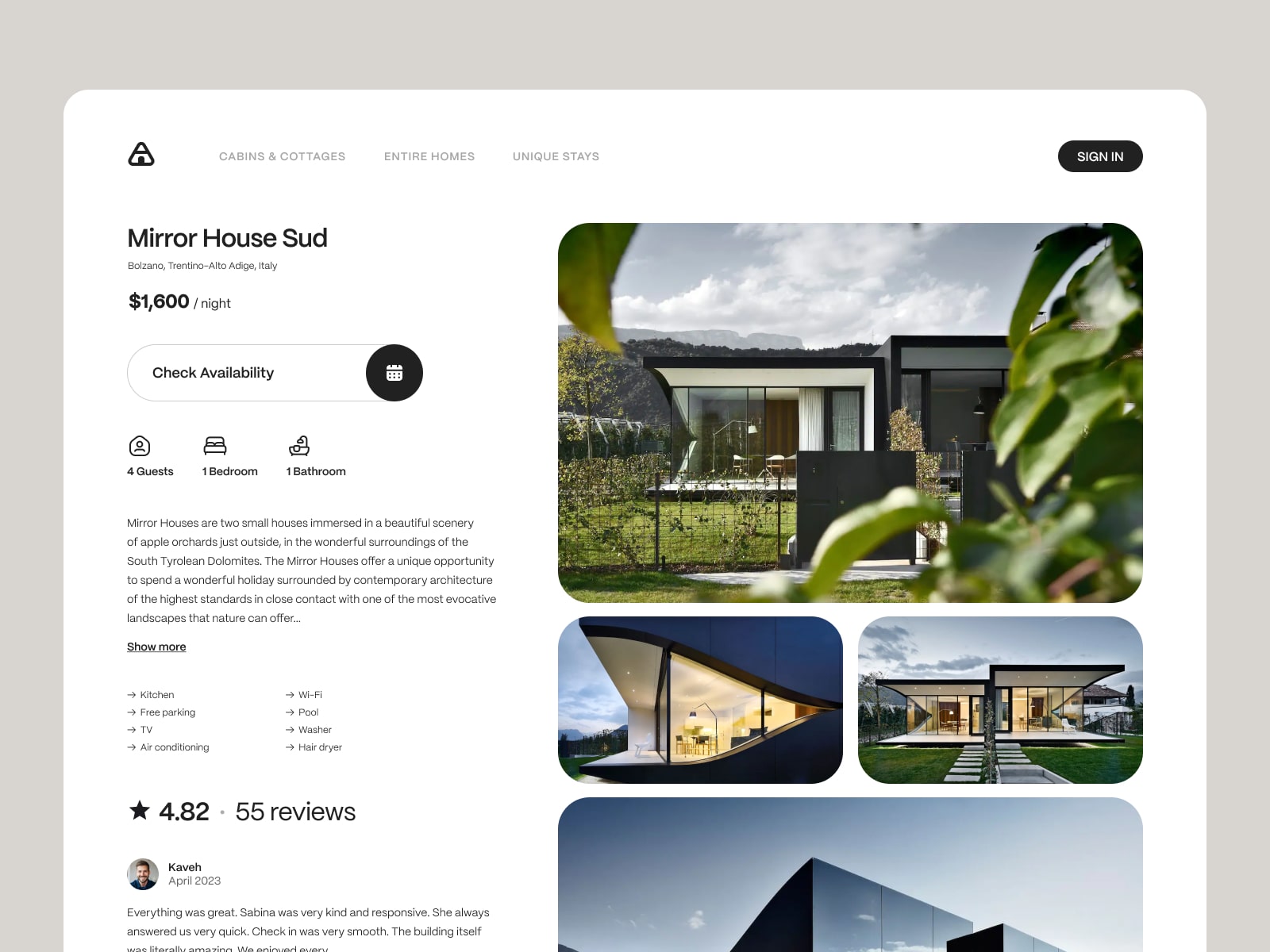
Rental Booking Service Website Design by Conceptzilla
FAQ Section
How Often Should Code Reviews Be Done?
They need to be a routine, continuous part of your development process. Not an afterthought or something you’ll get around to “when you have time.” Ideally, you’d review the code each time any team member creates a pull request (PR) or pushes code to the main branch. So every change, no matter how small, is reviewed for quality, consistency, and correctness before it’s committed to the common codebase.
What’s More Important: Speed or Code Quality?
Both code quality and speed are important, but they must coexist. Sacrificing one completely for the other almost always leads to disaster. Speed makes you competitive and responsive, and good software is stable and maintainable. The trick is to prioritize smartly, talk clearly, and use tools and processes to achieve both goals.
How to Convince Teams to Adopt Best Practices?
People are more likely to adopt something new if they understand why it matters. If you’re advocating for software engineering best practices, be the first to model them. Nothing convinces a team faster than seeing someone walk the walk. Developers aren’t the only ones who need convincing—stakeholders and managers also play a role. Speak their language by tying best practices to business outcomes and revenue.